binary tree python geeksforgeeks
Python Server Side Programming Programming. What is an ADT Write an algorithm to insert an element into BST.
Deletion In A Binary Tree Geeksforgeeks
1 3 2 Output.
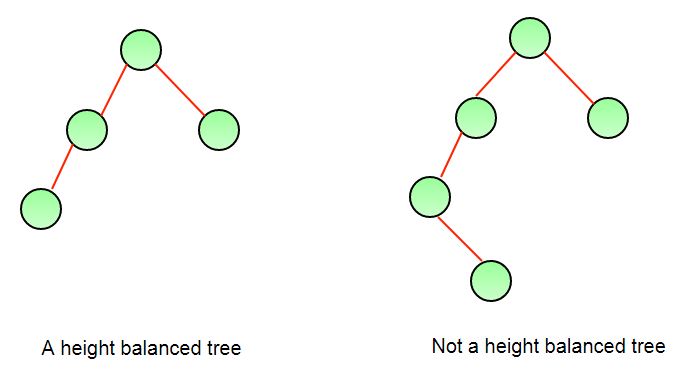
. Populate Inorder Successor for all nodes. Follow this answer to receive notifications. Complete the function cloneTree which takes root of the given tree as input parameter and returns.
If tree is not None. No need to read input or print anything. Binary tree python geeksforgeeks.
Find n-th node of inorder traversal. Given a binary tree find its height. Check if two nodes are cousins in a Binary Tree.
The tree will be created based on the height of each person. Note that Y and Z may be NULL or further nested. Tree was given in the form.
Find all possible binary trees with given Inorder Traversal. The value of the root node index would always be -1 as there is no parent for root. Construct the standard linked representation of Binary Tree from this array representation.
The subtree corresponding to the root node is the entire tree. A binary tree is a tree that has at most two children. Thus nodes of the binary tree will be printed as such 3 1 2.
Level Order Tree Traversal. The task is to complete two function serialize which takes the root node of the tree as input and stores the tree into an array and deSerialize which deserializes the array to the original tree and returns the root of it. The value of the root node index would always be -1 as there is no parent for root.
Check if all leaves are at same level. If binary search tree is empty create a new node and declare it as root if root is None. The array indexes are values in tree nodes and array values give the parent node of that particular index or node.
Given level order traversal of a Binary Tree check if the Tree is a Min-Heap. A tree is represented by a pointer to the topmost node in tree. Clone the given tree.
Given an array of size N that can be used to represents a tree. This article is contributed by Aditya Goel. Selfdata data selfleftChild None selfrightChild None def insertroot newValue.
A tree whose elements have at most 2 children is called a binary tree. A Binary tree is said to be balanced if the difference of height of left subtree and right subtree is less than or equal to 1. 10 20 30.
One node is marked as Root node. Note that the output to the program will always be a sorted sequence as we are printing the inorder traversal of a Binary Search Tree. Construct the standard linked representation of Binary Tree from this array representation.
Create a binary search tree in which each node stores the following information of a person. You dont need to read input or print anything. 2 1 3 Output.
Which of the following represents a valid binary tree. Also the smaller tree or the subtree in the left of the root node is called the Left sub-tree and that is on the right is called Right sub-tree. In a binary tree each node contains two children ie left child and right child.
The binary search tree is a special type of tree data structure whose inorder gives a sorted list of nodes or vertices. 0 5 7 6 4 1 3 9. Check if given Preorder Inorder and Postorder traversals are of same tree.
Replace each node in binary tree with the sum of its inorder predecessor and successor. A tree whose elements have at most 2 children is called a binary tree. Root BinaryTreeNodenewValue return root if newValue is less than value of data in root add it to left subtree and proceed recursively if.
The right subtree of a node contains only nodes with keys greater than the nodes key. Since each element in a binary tree can have only 2 children we typically name them the left and right child. Binary tree python geeksforgeeks.
Given a special binary tree having random pointers along with the usual left and right pointers. This code will run on Python 2 or 3 but on recent versions of Python 3 the traverse function can be written more compactly. Inorder Successor of a node in Binary Tree.
H is the height of the tree and this space is used implicitly for the recursion stack. Let us suppose we have a binary tree and we need to check if the tree is balanced or not. Python - Binary Tree.
A tree whose elements have at most two children is called a binary tree. Your task is to complete the function height which takes root node of the tree as input parameter and returns an integer denoting the height of the tree. Binary Tree Array implementation Given an array that represents a tree in such a way that array indexes are values in tree nodes and array values give the parent node of that particular index or node.
3 1 2 Explanation. Build a random binary tree. The left subtree of a node contains only nodes with keys lesser than the nodes key.
X Y Z indicates Y and Z are the left and right sub stress respectively of node X. The return type is cpp. The left and right subtree each must also be a binary search tree.
Consider the following nested representation of binary trees. Tree represents the nodes connected by edges. Binary Search Tree is a node-based binary tree data structure which has the following properties.
Given two binary trees check if the first tree is a subtree of the second one. Name age NID and height. It is a non-linear data structure.
1 2 3 Output. Your task is to complete the function Ancestors that finds all the ancestors of the key in the given binary tree. A subtree of a tree T is a tree S consisting of a node in T and all of its descendants in T.
Every node other than the root is associated with one parent node. Bst generates a random binary search tree and return its root node. How the search for an element in a binary search tree.
Therefore the time complexity of this approach is O N. Check if removing an edge can divide a Binary Tree in two halves. In Python we can directly create a BST object using binarytree module.
The node which is on the left of the Binary Tree is called Left-Child and the node which is the right is called Right-Child. Given a level K you have to find out the sum of data of all the nodes at level K in a binary tree. First case represents a tree with 3 nodes and 2 edges where root is 1 left child of 1 is 3 and right child of 1 is 2.
Construct the standard linked representation of given. If the tree is empty then value of root is NULL. Yield from traverse tree L yield tree D yield from traverse tree R Share.
The tree was cloned successfully. It has the following properties. The tree is a hierarchical Data Structure.
1. The subtree corresponding to any other node is called a proper subtree. If the tree is empty return 0.
Generate Complete Binary Tree In Such A Way That Sum Of Non Leaf Nodes Is Minimum Geeksforgeeks
Check Whether A Binary Tree Is A Complete Tree Or Not Set 2 Recursive Solution Geeksforgeeks
Introduction To Tree Data Structure Geeksforgeeks
Print All Nodes At Distance K From A Given Node Geeksforgeeks
Find Sum Of All Left Leaves In A Given Binary Tree Geeksforgeeks
Serialize And Deserialize A Binary Tree Geeksforgeeks
Tutorial On Binary Tree Geeksforgeeks
Construct A Binary Tree In Level Order Using Recursion Geeksforgeeks
Binary Tree Using Dstructure Library In Python Geeksforgeeks
Print Path Between Any Two Nodes In A Binary Tree Geeksforgeeks
Find Minimum Depth Of A Binary Tree Geeksforgeeks
Print Left View Of A Binary Tree Geeksforgeeks
Difference Between Binary Tree And Binary Search Tree Geeksforgeeks
How To Determine If A Binary Tree Is Height Balanced Geeksforgeeks
Deepest Left Leaf Node In A Binary Tree Iterative Approach Geeksforgeeks Youtube
Insertion In A Binary Tree In Level Order Geeksforgeeks
Pairwise Swap Leaf Nodes In A Binary Tree Geeksforgeeks
Check If Given Sorted Sub Sequence Exists In Binary Search Tree Geeksforgeeks